Front-End Framework for Artists
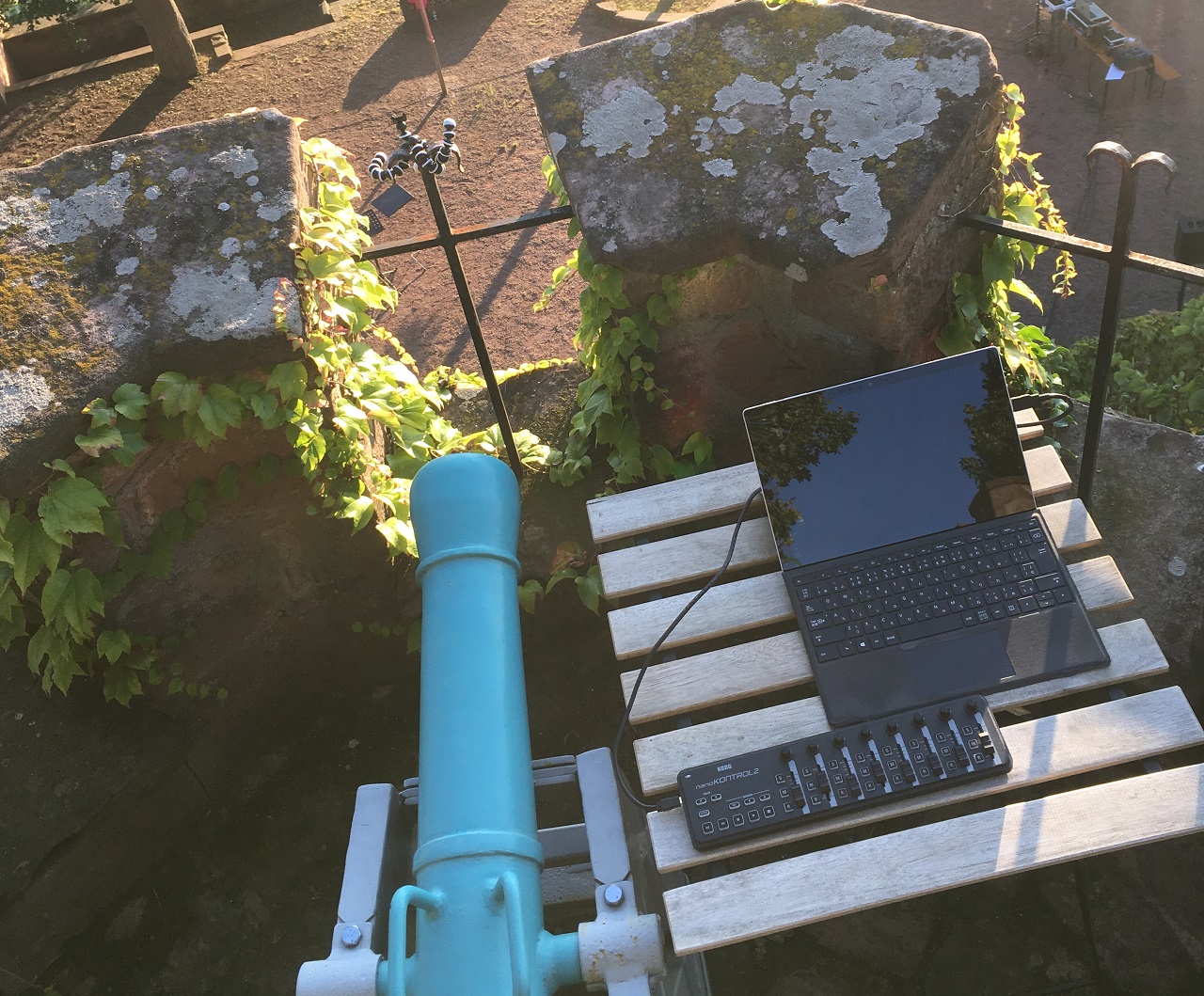
Update 23 Oct, 2021: 1) Added torus framework. 2) Now you can find examples on my repository and live page. These examples include Hydra and p5.js with choo, vue, alpine, mithril and torus.
Since I broke my finger a few days ago, I am killing time by watching tutorials, mainly on web front-end frameworks. There are famous ones like React and Vue, but also I got to know less popular frameworks. I have been checking out frameworks in order to know what is suitable for rapid prototyping, often ideal for creative coding. But first of all, does creative coding need a framework? Over the last few years, I have been using JavaScript libraries like p5.js and Hydra to create interactive web experiments. Nevertheless, it was around a year ago I started learning a framework, thanks to Olivia Jack, to create a responsive “single page app” with herself and a few others for Hydra meetup. Since then, I learned the power of front-end frameworks - rather than making ad-hoc interactions in a JQuery style (manipulating DOM manually, same for vanilla JavaScript and p5.js), which tend to end up with a spaghetti code, using a framework enables us to easily create page transitions, and what is more, to reuse old code for new projects.
In this article, I would like to compare some of the popular frameworks with a creative use in mind. I roughly rated from three aspects: learning curve if the framework is easy to learn; hackable if it allows acrobatic tricks; maintainable if the code is easy to reuse later. Also I added if the framework works with <script>
tag import or not because most of the frameworks are not intended to be used as such. For every framework, I attached “hello world” script to give you a bit of the idea of how the code looks like. Also there is a link to p5.js Editor for an example of how to work with p5.js for frameworks that support import with <script>
tag. The example shows a simple demo of assigning text input field and a slider to affect the canvas.
React
- learning curve: ❌
- hackable: ❌
- maintainable: ⭕
- works with script import: ❌
Hello World
1
2
3
4
ReactDOM.render(
<h1>Hello, world!</h1>,
document.getElementById('root')
);
React from Facebook is definitely the most popular framework in the industry. Only recently I started using it for an artistic project as I inherited the code already written in React (Hooks). I found it very structured at the cost of being verbose - for example, you need to define different components and pages. But the largest downside, in my opinion, is how the state is organized, and it does not allow you to do acrobatic tricks like storing everything in a global variable, like some might do in Processing or openFrameworks for a quick fix before an exhibition or a performance. Also, how the page is rendered is unlike draw
loops in creative coding tools, and it may take a while to get the hang of it. The other problem is that the code always has to be compiled through a webpack pipeline. This means always npm and a backend server is required to develop a React project, and you cannot simply start coding on p5.js Editor or Codepen.
Its rigid structure makes it difficult to add tools like p5.js - you may need to import a package like react-p5, which, of course, requires the instance mode.
Note that there are mainly two versions, original React and React Hooks, and I highly recommend Hooks if you decide to use React for creative applications for some reason. It is less wordy although useState
and useEffect
syntaxes are quite confusing at first.
Choo
- learning curve: 🔺
- hackable: 🔺
- maintainable: ⭕
- works with script import: ⭕
Hello World
1
2
3
4
5
var app = choo()
var main = function () {
return html`<div>choo animals</div>`
}
app.mount('div')
Example on p5.js Editor
https://editor.p5js.org/micuat/sketches/rS8lvWxpH
Choo is a framework, which Olivia told me, and used for the webpage above. The official documentation is very friendly and easy to begin with. While they encourage the use of budo
to bundle the script, you can also import the code with <script>
tag (reference). While it remains as one of my favorite frameworks, there are two downsides in my opinion. One is that writing tags with html
template literals (backticks) can get messy especially on an online editor that you cannot customize, like glitch. Another problem is that the idea of emitter and store are quite confusing; on one hand you can do acrobatic tricks by emitting commands from one to another, but if you do not understand the lifecycle well, you can easily stuck in infinite re-rendering loop.
I made choo-p5js-template as a starter kit to use p5.js with choo.
Vue
- learning curve: ❌
- hackable: 🔺
- maintainable: ⭕
- works with script import: ⭕
Hello World
<div id="app">
{{ message }}
</div>
1
2
3
4
5
6
var app = new Vue({
el: '#app',
data: {
message: 'Hello Vue!'
}
})
Example on p5.js Editor
https://editor.p5js.org/micuat/sketches/fz3W-BuDI
Vue is one of the big three frameworks (I am not sure if you add Angular or Svelte in big three these days, though). In fact, this is the first framework that I tried to learn, but I gave up and I only use it occasionally when I want to bind values between the script and the HTML. As you can see in their documentary, the community is nerdy and that is reflected by their design philosophy (by the way, I found the documentary quite interesting especially highlighting the community). Contrary to the previous frameworks above, Vue is a combination of HTML (file) and JavaScript while the previous two basically has HTML tags inside the script. Simple bindings work surprisingly easy - for example, if you change the message
value in the example above, that will be reflected on the rendered HTML. However, if you plan to add more complexity, the binding between HTML and JavaScript can get very confusing; there are keywords like data
, computed
, watch
and methods
, and if you want to create a complex interaction, you need to fully understand its lifecycle.
The framework can be used as a library, i.e., you can import with <script>
tag, which is an upside of the library. In fact, we use it for GlitchMe webpage. Nevertheless, if you plan to use Vue as a library, I recommend you to take a look at Alpine in the next section.
Alpine
- learning curve: ❌
- hackable: 🔺
- maintainable: 🔺
- works with script import: ⭕
Hello World
1
2
3
4
<div x-data="{ message: 'hello world!' }">
<div x-text="message">
</div>
</div>
Example on p5.js Editor
https://editor.p5js.org/micuat/sketches/TDcwOji5h
Alpine is a lightweight library inspired by Vue; therefore, the design philosophy is quite similar (and nerdy). It is made to be used as <script>
import in mind, and as you can see in the example, it encourages inline JavaScripts in HTML. I immediately fell in love with this acrobatic nature. I did try it to create a clone of GlitchMe, which I did manage, but I found that the error messages are not friendly (or you don’t even know what DOM is giving an error).
In case you are already familiar with Vue but not willing to set up webpack to make, for example, an interactive page with p5.js, Alpine can be a good option. However, I do not recommend this library as a first framework for creative coders to jump in.
Svelte
- learning curve: ⭕
- hackable: ❌
- maintainable: ⭕
- works with script import: ❌
Hello World
<script>
let name = 'world';
</script>
<h1>Hello {name}!</h1>
Svelte is undeniably the cleanest front-end framework - in one file, you can define script, html and style, and unlike Vue, there is no complex data structure. I really love it, and I recommend it if you are planning to make a webpage with more than just a few interactions. I used it for KHMN Festival and Netze Open pages and I absolutely loved the experience. And the tutorial is very clean and helpful.
Downsides are 1) components and states can get a bit wordy and 2) you always need to setup a compilation pipeline. The first point may be due to the lack of my experience, but certainly Svelte is a rigid framework that does not allow use of global variables and such. In fact, I could not make it work with a library like Hydra that exposes functions as global variables. The latter point is more critical for creative applications; as Svelte is a compiler, you always need to set up a pipeline like webpack or rollup to compile. Therefore, it does not run on p5.js Editor, for example. I see its standpoint like openFrameworks in creative coding.
If you want to combine Svelte with p5.js, you might want to take a look at p5-svelte (requires instance mode).
Mithril
- learning curve: ⭕
- hackable: ⭕
- maintainable: 🔺
- works with script import: ⭕
Hello World
1
2
3
var root = document.body
var message = "My first app"
m.render(root, m("h1", message))
Example on p5.js Editor
https://editor.p5js.org/micuat/sketches/-tSm0KGDE
At last, I want to introduce Mithril, which is a lightweight framework and takes quite a different approach from others. Unlike the frameworks we have seen, there is no HTML tags; in fact, the function call m('div', text)
generates a <div>
with text
as content (technically not, but let’s say it does), similar to createElement
in vanilla JavaScript or p5.js. Nevertheless, if variable text
, the content of m()
, changes, Mithril detects the change and renders the updated HTML.
I ported GlitchMe in Mithril and I enjoyed the experience as all the data can be global, and I can use bad practices in web development to make quick and dirty code. The downsides are that it can be quick and dirty, and nested tags can become very lengthy, for example in the code:
1
2
3
4
5
6
7
8
9
10
11
12
showModal &&
m("div.modal-mask", [
m("div.modal-wrapper", [
m("div.modal-container", [
m("div.modal-header", [m("h3", "GlitchMe")]),
m(
"div.modal-body",
showModal === "howto"
? [
m(
"p",
...
Torus
- learning curve: ⭕
- hackable: ⭕
- maintainable: ⭕
- works with script import: ⭕
Hello World
1
2
3
4
5
6
7
8
9
10
11
class App extends Torus.StyledComponent {
init() {
this.message = "Hello!";
}
compose() {
return jdom`<main>
<h1>${this.message}</h1>
</main>`;
}
}
document.body.appendChild(new App().node);
Example on p5.js Editor
https://editor.p5js.org/micuat/sketches/JBBkwVZsy
Torus is another lightweight framework; while it is not as popular as other frameworks, it has a clean structure while allowing ad-hoc expressions using template literals (jdom
in the example above). It looks like a combination of Svelte (data, style and html in one place), Vue (data structure) and Choo (inline html), yet lightweight and allowing <script>
import.
Conclusions
As you can see, every framework has pros and cons, but if I pick one for creative coding, I would recommend Mithril and Torus. I have not used them extensively so my opinion may change, but I see that the frameworks go well with hacky approach of tools like p5.js and Hydra while with other frameworks, you might face problems with scopes and states. But first, I suggest looking into those with a p5.js example (Choo, Vue, Alpine, Mithril, Torus) and tweak the example to see if it feels natural for you. If you are already familiar with npm, Svelte may be a good choice, too.